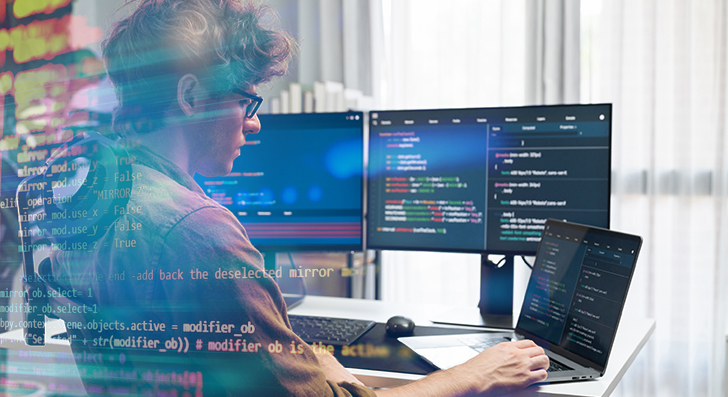
Scalability usually means your software can tackle expansion—far more customers, additional knowledge, and a lot more site visitors—without having breaking. As being a developer, setting up with scalability in your mind saves time and strain afterwards. Right here’s a transparent and functional manual to help you start off by Gustavo Woltmann.
Style for Scalability from the beginning
Scalability is not some thing you bolt on afterwards—it should be aspect of one's approach from the beginning. Lots of programs are unsuccessful if they develop rapid since the first style can’t cope with the extra load. Being a developer, you need to Feel early about how your technique will behave stressed.
Commence by building your architecture to become versatile. Stay clear of monolithic codebases in which all the things is tightly linked. In its place, use modular design and style or microservices. These designs crack your app into smaller sized, impartial pieces. Every module or provider can scale on its own with no influencing the whole method.
Also, think of your databases from working day one. Will it want to manage a million end users or merely 100? Pick the right kind—relational or NoSQL—determined by how your facts will mature. Plan for sharding, indexing, and backups early, Even though you don’t will need them nonetheless.
Another essential stage is in order to avoid hardcoding assumptions. Don’t publish code that only performs underneath latest situations. Think of what would come about When your consumer base doubled tomorrow. Would your app crash? Would the database slow down?
Use design styles that help scaling, like message queues or occasion-driven systems. These help your application tackle extra requests devoid of finding overloaded.
When you build with scalability in your mind, you are not just getting ready for success—you're reducing upcoming problems. A very well-planned process is simpler to keep up, adapt, and grow. It’s better to get ready early than to rebuild later on.
Use the proper Databases
Picking out the proper databases is really a key Element of constructing scalable programs. Not all databases are built a similar, and utilizing the Incorrect you can sluggish you down as well as trigger failures as your application grows.
Start off by knowing your data. Could it be extremely structured, like rows in a desk? If yes, a relational databases like PostgreSQL or MySQL is a great suit. They are potent with associations, transactions, and regularity. They also aid scaling strategies like read replicas, indexing, and partitioning to manage more traffic and knowledge.
In case your facts is more versatile—like person activity logs, product or service catalogs, or documents—look at a NoSQL choice like MongoDB, Cassandra, or DynamoDB. NoSQL databases are better at dealing with significant volumes of unstructured or semi-structured information and might scale horizontally more very easily.
Also, take into consideration your study and produce patterns. Have you been performing a great deal of reads with much less writes? Use caching and read replicas. Have you been dealing with a major create load? Investigate databases which will handle large publish throughput, or perhaps function-dependent details storage systems like Apache Kafka (for short-term knowledge streams).
It’s also clever to Imagine ahead. You may not require Highly developed scaling features now, but choosing a database that supports them indicates you won’t want to change later on.
Use indexing to speed up queries. Keep away from unwanted joins. Normalize or denormalize your facts dependant upon your entry designs. And constantly watch databases effectiveness when you improve.
Briefly, the appropriate databases will depend on your application’s composition, velocity desires, And just how you be expecting it to increase. Just take time to choose wisely—it’ll save a lot of hassle afterwards.
Optimize Code and Queries
Speedy code is essential to scalability. As your application grows, each and every tiny delay provides up. Inadequately prepared code or unoptimized queries can slow down overall performance and overload your system. That’s why it’s vital that you Develop efficient logic from the beginning.
Start off by creating clean, very simple code. Prevent repeating logic and remove anything avoidable. Don’t select the most complicated Answer if a straightforward just one operates. Keep your features brief, concentrated, and simple to check. Use profiling equipment to search out bottlenecks—sites in which your code takes much too prolonged to run or works by using an excessive amount memory.
Up coming, look at your databases queries. These often sluggish matters down in excess of the code itself. Ensure that Each and every query only asks for the info you really need. Keep away from Choose *, which fetches anything, and as an alternative find unique fields. Use indexes to hurry up lookups. And avoid executing too many joins, In particular throughout large tables.
In case you notice precisely the same details getting asked for time and again, use caching. Retail store the outcome quickly utilizing equipment like Redis or Memcached so you don’t must repeat highly-priced operations.
Also, batch your database operations any time you can. Rather than updating a row one after the other, update them in groups. This cuts down on overhead and helps make your application extra efficient.
Remember to examination with substantial datasets. Code and queries that work fantastic with one hundred documents may well crash if they have to deal with one million.
Briefly, scalable applications are speedy apps. Keep your code tight, your queries lean, and use caching when required. These actions aid your application keep clean and responsive, whilst the load boosts.
Leverage Load Balancing and Caching
As your app grows, it has to handle much more customers and even more targeted traffic. If almost everything goes by just one server, it can promptly turn into a bottleneck. That’s the place load balancing and caching can be found in. These two instruments enable maintain your app quickly, stable, and scalable.
Load balancing spreads incoming traffic throughout various servers. In lieu of just one server doing all the do the job, the load balancer routes people to diverse servers dependant on availability. What this means is no solitary server gets overloaded. If a person server goes down, the load balancer can send out traffic to the others. Applications like Nginx, HAProxy, or cloud-dependent answers from AWS and Google Cloud make this easy to arrange.
Caching is about storing knowledge temporarily so it might be reused quickly. When people request the same facts once again—like a product site or even a profile—you don’t have to fetch it within the database every time. You may serve it within the cache.
There are 2 frequent types of caching:
one. Server-side caching (like Redis or Memcached) merchants information in memory for rapid accessibility.
two. Client-aspect caching (like browser caching or CDN caching) stores static documents close to the consumer.
Caching cuts down database load, improves pace, and will make your app additional effective.
Use caching for things which don’t change typically. And always be sure your cache is current when info does transform.
In short, load balancing and caching are basic but powerful equipment. Collectively, they assist your application manage additional users, remain rapidly, and Get better from issues. If you intend to improve, you need the two.
Use Cloud and Container Instruments
To build scalable applications, you will need tools that allow your app increase conveniently. That’s where cloud platforms and containers are available in. They provide you overall flexibility, decrease setup time, and make scaling Considerably smoother.
Cloud platforms like Amazon World-wide-web Services (AWS), Google Cloud Platform (GCP), and Microsoft Azure let you rent servers and providers as you will need them. You don’t have to buy hardware or guess long term capability. When site visitors will increase, it is possible to insert far more methods with just a couple clicks or mechanically working with car-scaling. When website traffic drops, you may scale down to economize.
These platforms also present services like managed databases, storage, load balancing, and security applications. You could deal with making your application as opposed to handling infrastructure.
Containers are An additional important tool. A container offers your application and every little thing it must run—code, libraries, configurations—into one particular unit. This makes it simple to maneuver your application among environments, from your notebook on the cloud, without having surprises. Docker is the most popular Software for this.
Whenever your app takes advantage of many containers, equipment like Kubernetes assist you to regulate them. Kubernetes handles deployment, scaling, and Restoration. If 1 section of the app crashes, it restarts it immediately.
Containers also enable it to be simple to separate portions of your app into products and services. It is possible to update or scale components independently, and that is great for general performance and dependability.
In short, employing cloud and container tools suggests you are able to scale rapid, deploy effortlessly, and Get better speedily when issues transpire. If you would like your application to grow without having restrictions, begin working with these resources early. They help save time, reduce threat, and assist you stay focused on making, not fixing.
Check All the things
In the event you don’t keep an eye on your application, you gained’t know when factors go Completely wrong. Monitoring aids the thing is how your application is performing, spot troubles early, and make superior decisions as your app grows. It’s a crucial Section of setting up scalable systems.
Commence by tracking standard metrics like CPU use, memory, disk House, and reaction time. These inform you how your servers and expert services are accomplishing. Tools like Prometheus, Grafana, Datadog, or New Relic will help you gather and visualize this knowledge.
Don’t just watch your servers—watch your application much too. Regulate how much time it takes for users to load pages, how often errors happen, and exactly where they happen. Logging resources like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly will help you see what’s happening inside your code.
Set up alerts for critical troubles. By way of example, When your response time goes over a limit or a service goes down, you should get notified immediately. This allows you deal with difficulties rapidly, usually just before customers even notice.
Checking can be beneficial whenever you make changes. For those who deploy a different attribute and find out a spike in mistakes or slowdowns, you can roll it again ahead of it leads to real problems.
As your app grows, traffic and facts boost. With out checking, you’ll skip indications of difficulties till it’s much too late. But with the best resources set up, you remain on top of things.
In brief, checking aids you keep the app click here responsible and scalable. It’s not pretty much spotting failures—it’s about being familiar with your program and ensuring that it works perfectly, even under pressure.
Remaining Ideas
Scalability isn’t only for big firms. Even small applications have to have a powerful Basis. By creating thoroughly, optimizing wisely, and using the suitable resources, you may build apps that mature smoothly with no breaking stressed. Begin compact, Believe massive, and build intelligent.